For our ICM final project my partner and I decided to combine it with PComp and make an interface for the game we wanted to develop.
We wanted to make a game called Tagman that is a combination of Pac-Man and Tag. In essence it would be a Pac-Man game played in real life but with multiple players.
Due to time, budget and logistic constraints we only made two vests for players and to keep it a little more simple we turned the game into three mini games of tag where each character would tag another and the mini games would be random.
The interface we designed is meant to explain the game to the players and what the color of the LEDs on the vests mean.
For the structure of the code I decided to make each "screen" be a separate file within my sketch and within a function that could be called forward at the right time. I learned more about case switch and how that could help play my sketches in sequence. I also learned how to set a timer for my sketches to skip to the next one.
CODE for MAIN SKETCH:
let b = 200;
let gameLevel = 0;
let gameTimer = 0;
let gameCounter = 0;
let eatSound;
let pacmanSound;
let waitingSound;
let tagSound;
function preload() {
myFont = loadFont('Pixeled.ttf');
eatSound = loadSound('waka.mp3');
pacmanSound = loadSound('PacmanThemeRemix.mp3');
tagSound = loadSound('GameOver.wav');
}
function setup() {
createCanvas(windowWidth, windowHeight);
}
function draw() {
// background(0);
switch (gameLevel) {
case 0:
gameLevel = start();
break;
case 1:
gameLevel = instructions();
break;
case 2:
gameLevel = miniGames();
break;
case 3:
loading();
break;
case 4:
greenTag();
break;
case 5:
orangeTag();
break;
case 6:
blueForAll();
break;
case 7:
gameOver();
break;
}
blinking();
if (gameCounter > 4) {
gameOver();
}
}
I start the game with a Title screen and some theme music. I really wanted to animate the text but every time I tried it it would make my code freak out so I stopped.
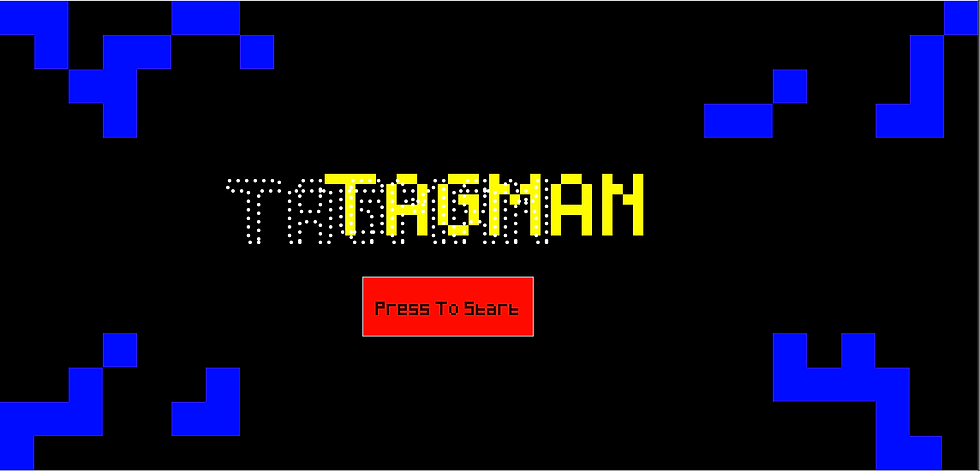
There's a button that you're meant to press to start the game.
CODE:
gameLevel = 0;
let button1;
let myFont;
function start() {
// button4.remove();
pacmanSound.play();
noStroke();
background(0);
textAlign(CENTER);
textSize(60);
fill('#FFFD00');
textFont(myFont);
text("TAGMAN", width / 2, height / 2);
var points = myFont.textToPoints("TAGMAN", width / 4.3, height / 1.94, 60);
for (let i = 0; i < points.length; i++) {
let pt = points[i];
stroke(255);
strokeWeight(4);
point(pt.x, pt.y);
}
let col = color(255, 10, 0);
button1 = createButton('Press To Start');
button1.position(width / 2.7, height / 1.7);
button1.mousePressed(instructions);
button1.style('font-size', '13px')
button1.style("font-family", "Pixeled");
button1.size(200, 70);
button1.style('background-color', col);
noStroke();
fill('#000DFF');
rectMode(CENTER);
rect(20, 20, 40, 40);
rect(60, 20, 40, 40);
rect(60, 60, 40, 40);
rect(100, 100, 40, 40);
rect(140, 100, 40, 40);
rect(140, 60, 40, 40);
rect(180, 60, 40, 40);
rect(140, 140, 40, 40);
rect(20, (height - 20), 40, 40);
rect(20, (height - 60), 40, 40);
rect(60, (height - 60), 40, 40);
rect(100, (height - 60), 40, 40);
rect(100, (height - 100), 40, 40);
rect(140, (height - 140), 40, 40);
rect((width - 20), 20, 40, 40);
rect((width - 60), 60, 40, 40);
rect((width - 60), 100, 40, 40);
rect((width - 60), 140, 40, 40);
rect((width - 100), 140, 40, 40);
rect((width - 20), (height - 20), 40, 40);
rect((width - 60), (height - 20), 40, 40);
rect((width - 100), (height - 20), 40, 40);
rect((width - 100), (height - 60), 40, 40);
rect((width - 100), (height - 100), 40, 40);
rect((width - 140), (height - 100), 40, 40);
rect((width - 180), (height - 100), 40, 40);
rect((width - 220), (height - 100), 40, 40);
rect((width - 220), (height - 140), 40, 40);
rect((width - 140), (height - 140), 40, 40);
rect(220, 20, 40, 40);
rect(260, 20, 40, 40);
rect(300, 60, 40, 40);
rect(220, (height - 60), 40, 40);
rect(260, (height - 60), 40, 40);
rect(260, (height - 100), 40, 40);
rect((width - 220), 100, 40, 40);
rect((width - 260), 140, 40, 40);
rect((width - 300), 140, 40, 40);
}
CODE for INSTRUCTIONS:
gameLevel = 1;
function instructions() {
button1.remove();
background(0);
textAlign(CENTER, CENTER);
let col = color(255, 10, 0);
textSize(50);
textFont('Pixeled');
fill(255, 255, 46);
text('INSTRUCTIONS', 360, 80);
textAlign(LEFT);
fill(255, 20, 20);
textSize(14);
text('-YOU HAVE 30 SECS TO THINK STRATEGY', 40, 150);
fill(255, 255, 46);
text('-YELLOW MEANS PACMAN.', 40, 200);
fill(239, 12, 239);
text('-PINK MEANS GHOST.', 40, 250);
fill(255, 20, 20);
text('-PAY ATTENTION TO THE MINI GAMES, THERE ARE 3.', 40, 300);
fill(70, 183, 9);
text('-GREEN MEANS PACMAN TAGS GHOST', 40, 350);
fill(255, 115, 0);
text('-ORANGE MEANS GHOST TAGS PACMAN', 40, 400);
fill(34, 0, 237);
text('-BLUE means FREE for ALL', 40, 450);
button2 = createButton('CONTINUE >');
button2.position(width / 1.5, height / 1.2);
button2.mousePressed(miniGames);
button2.style('font-size', '13px')
button2.style("font-family", "Pixeled");
button2.size(150, 50);
button2.style('background-color', col);
}
CODE FOR MIN GAMES:
gameLevel = 2;
function miniGames(){
// waitingSound.play();
button2.remove();
noStroke();
background(0);
textAlign (CENTER, CENTER);
textSize(50);
textFont('Pixeled');
fill(255, 255, 46);
text('MINI GAMES', (width/2), 80);
fill('#8FFF17');
rectMode(CENTER);
rect((width/2), 180, width, (height/5));
fill('#FF9100');
rect((width/2), 280, width, (height/5));
fill('#000DFF');
rect((width/2), 380, width, (height/5));
fill(0);
textSize(20);
text('GREEN MEANS PACMAN TAG GHOST', (width/2), (height/5+80));
text('ORANGE MEANS GHOST TAG PACMAN', (width/2), (height/4+160));
text('BLUE MEANS FREE FOR ALL', (width/2), (height/5+280));
let col = color(255, 10, 0);
button3 = createButton('START TAGGING >');
button3.position(width/3, height/1.15);
button3.mousePressed(function() {
loading();
gameTimer=0;
});
button3.style('font-size', '13px')
button3.style("font-family", "Pixeled");
button3.size(250, 50);
button3.style('background-color', col);
}
After you read and understand the instructions then the game sorts through and picks a Mini Game at random.
CODE for LOADING SCREEN:
gameLevel = 3;
function loading(){
gameTimer+=deltaTime;
if( gameTimer>100) {
gameLevel= int(random(4,7));
gameTimer=0;
}
button3.remove();
noStroke();
background(0);
textAlign (CENTER, CENTER);
textFont('Pixeled');
fill(255, 255, 46);
textSize(40);
text('LOADING...', (width/2*1.1), (height/3*2.2));
ellipse((width/2), (height/3*1.3), 190, 190);
fill(0);
triangle((width/2), 230, (width/2+100), 270, (width/2+100), 160);
// fill(255, 255, 46);
// rectMode(CENTER);
// rect((width-20), (height-20), 40, 40);
}
The LEDs on the vest reflect the mini game to be played and the character assigned to each player.
CODE for TAG PACMAN:
gameLevel=5;
function orangeTag(){
gameTimer+=deltaTime;
if( gameTimer>500) {
gameLevel= int(random(4,7));
gameTimer=0;
gameCounter++
}
background('#FF9100');
fill(0);
textAlign (CENTER, CENTER);
textSize(45);
textFont('Pixeled');
text('TAG PACMAN', (width/2), (height/2));
}
CODE for TAG GHOST:
gameLevel=4;
function greenTag(){
gameTimer+=deltaTime;
if( gameTimer>500) {
gameLevel= int(random(4,7));
gameTimer=0;
gameCounter++
}
background ('#8FFF17');
// fill(255, 255, 46);
// rectMode(CENTER);
// noStroke();
// rect((width-20), (height-20), 40, 40);
fill(0);
textAlign (CENTER, CENTER);
textSize(45);
textFont('Pixeled');
text('TAG GHOSTS', (width/2), (height/2));
}
CODE for FREE FOR ALL:
gameLevel=6;
function blueForAll(){
gameTimer+=deltaTime;
print ( gameTimer);
if( gameTimer>500) {
gameLevel= int(random(4,7));
print( gameLevel);
gameTimer=0;
gameCounter++
}
background('#000DFF');
fill(0);
textAlign (CENTER, CENTER);
textSize(45);
textFont('Pixeled');
text('FREE FOR ALL', (width/2), (height/2));
}
Once all the mini games are played then the game ends and can be restarted.
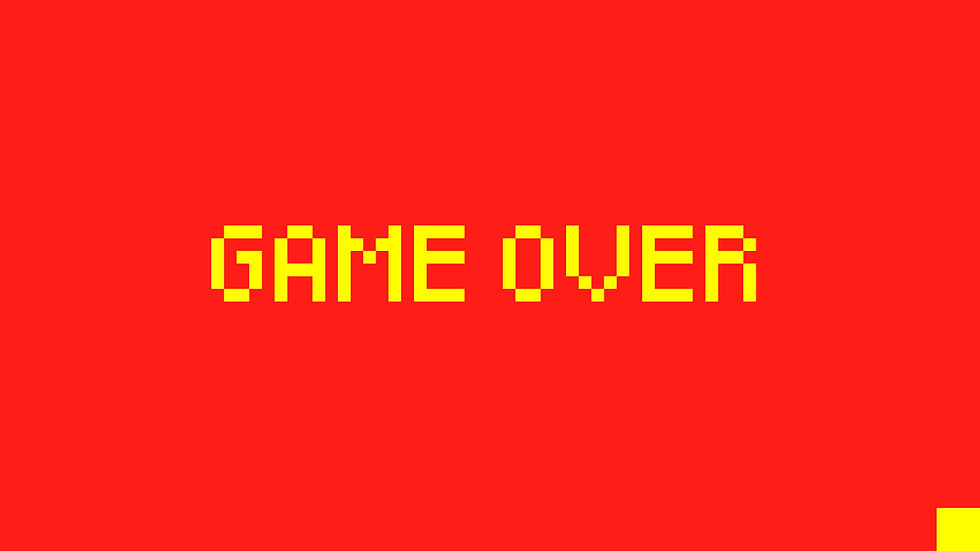
CODE for GAME OVER:
gameLevel = 7;
function gameOver() {
let col = color(255, 10, 0);
background(col);
fill(255, 255, 46);
// rectMode(CENTER);
// noStroke();
// rect((width-20), (height-20), 40, 40);
textAlign(CENTER, CENTER);
textSize(60);
textFont('Pixeled');
text('GAME OVER', (width / 2), (height / 2));
button4 = createButton('RESTART');
button4.position(width / 2.6, height / 1.6);
button4.mousePressed(function() {
gameLevel = 0;
gameCounter = 0;
start();
// gameLevel = 1;
})
button4.mouseClicked(button4.remove)
button4.style('font-size', '13px')
button4.style("font-family", "Pixeled");
button4.size(150, 50);
pacmanSound.stop();
}
I wanted to add things to the sketch (like animations) and fix the stuff that doesn't work (switch the music with each sketch, and connect our sketch to bluetooth so it can communicate with our wearables) but was unable to because of technical difficulties and some time constraints that weren't foreseen. I will keep working on it because our project is meant to be in the Winter Show and I'd like to perfect it for then.
I believe what made this project so difficult is the fact that we couldn't find much research on how to combine wearables with bluetooth and a sketch so we didn't have that many examples to work with. We also had the added difficulty that there are at least 700 bluetooth connected devices on the floor at any given time which interfered with our signal.
I honestly think I've become a lot more comfortable with code compared to how I was at the beginning of the semester. I think it is very necessary for the kind of work we do at ITP but it shouldn't be taken lightly.
Comments