Final Song
- sal5014
- May 6, 2020
- 5 min read
It has been a really cool journey with this project. Helen and I were a little concerned that our nonexistent knowledge of music would be really detrimental to our project's quality. However we feel we've learned a lot and are fairly proud of what we've accomplished.
OUR PROJECT
We wanted to make an instrument that worked by sensing distance. We used a Theremin as a concept base. Because we aren't musicians we wanted to try to make some presets to make playing easier regardless of your level. We made several iterations with different interactions and designs.
The way it works up until now is: one sensor plays notes in C4 diatonic scale and the other plays them in C5. The potentiometer regulates and changes the scales. We tried using the potentiometer to change octaves with 12 note series like a piano as well so this control would depend on the instrument selected on the synth since some instruments have a wider range of notes than others.
OUR INSTRUMENT
We redesigned the instrument since last week to make it more compact and perhaps a more elegant implementation. Our execution right now is not the ideal since some of the materials weren't readily available to us. Ideally all our components would fit inside the cylinder with only the power cable coming out.
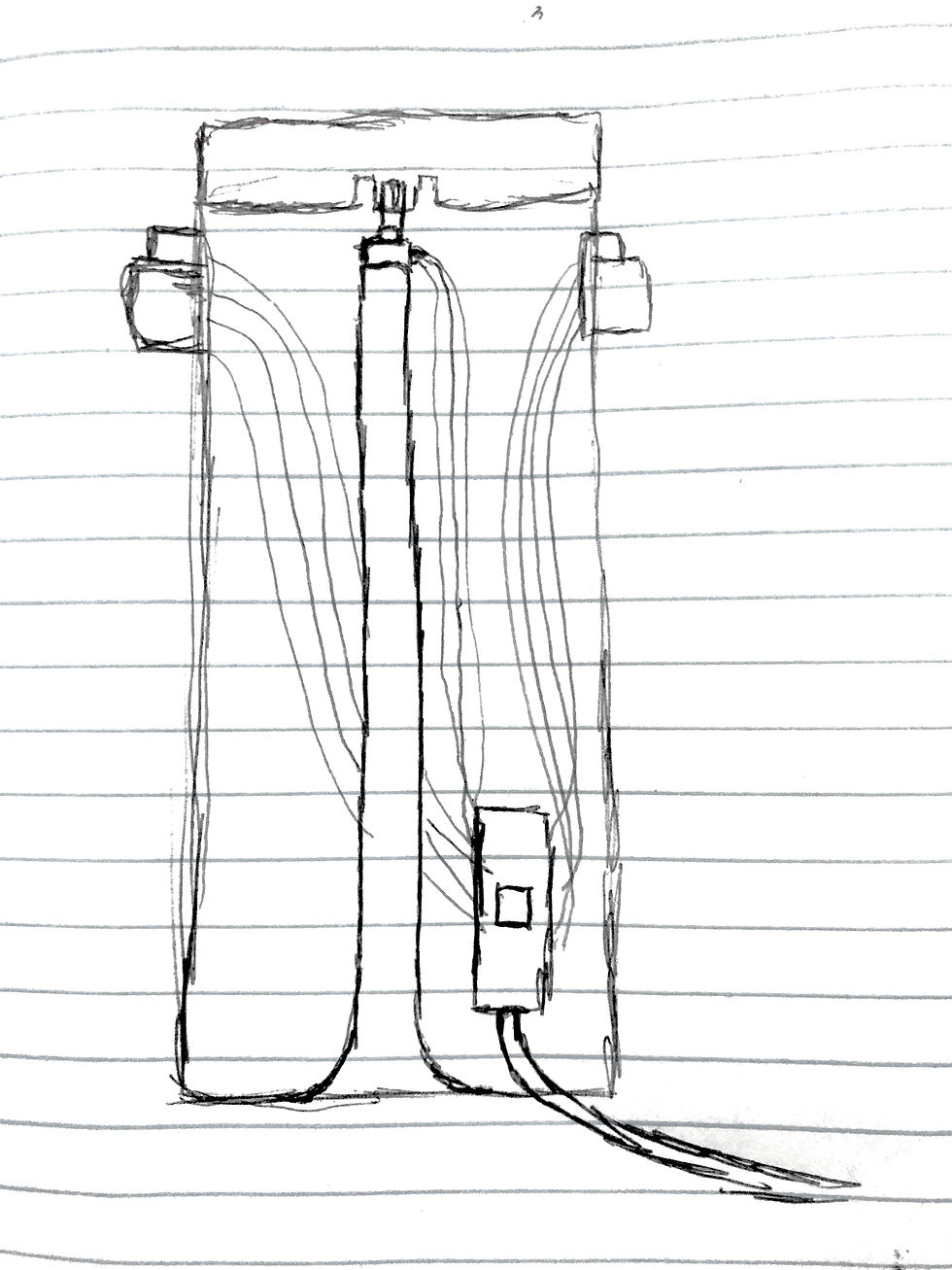
The reason they don't fit at the moment is because we used jumper cables that take up space and the tube in the middle that holds the potentiometer is too wide. Ideally we would use a sturdier and thinner material like wood to hold the potentiometer and the circuit would be soldered to take up less space.
We're proud of our knob though and we're pretty happy with. how it works. Here are some pictures and videos of the process and some of the finished and the "ideal" final.
OUR CIRCUIT
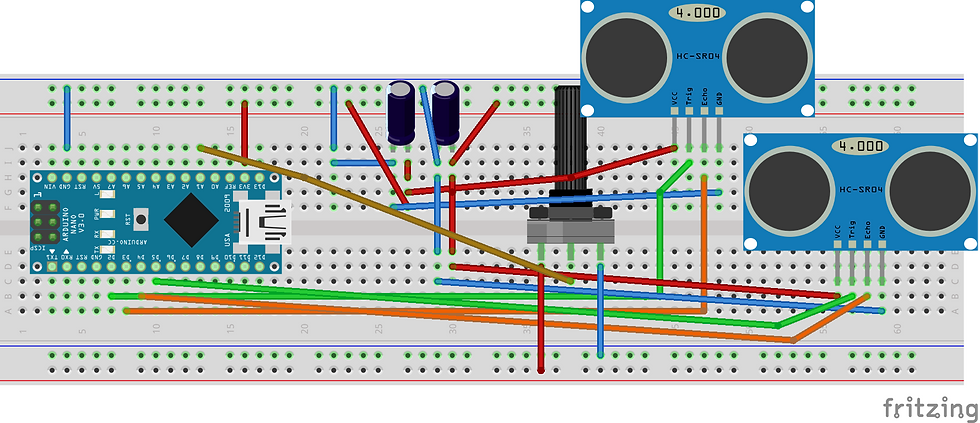
OUR CODE
//Sound project #include <MIDIUSB.h> #include <pitchToNote.h> int scale = 0; byte baseNote = 60; byte lastnote = 0; byte componot = 72; byte lastnote2 = 0; int trigPin1 = 4; int echoPin1 = 3; int trigPin2 = 2; int echoPin2 = 1; int lastDistance = 0; int lastDistance1 = 0; int dx; int dy; int sx; void setup() { // put your setup code here, to run once: Serial.begin (9600); pinMode(trigPin1, OUTPUT); pinMode(echoPin1, INPUT); pinMode(trigPin2, OUTPUT); pinMode(echoPin2, INPUT); } void loop() { // put your main code here, to run repeatedly: byte note = baseNote; byte comp= componot; long duration1, distance1; long duration2, distance2; int scale, sx, dx, dy; // This a variable resistor that could be replaced for a encoder or any knob analog interface to select the octave or scale of the midi instrument // This code is mapped for a flute, so it do not have many escale options as other more complex instruments may have scale = analogRead(A1); // Each value from the mapping code will give a start note for the scale or octave sx = map(scale, 70, 1000, 0, 4); if (sx == 1) { baseNote = 36; componot =48; } if (sx == 2) { baseNote = 48; componot=60; } if (sx == 3) { baseNote = 60; componot=72; } if (sx == 4) { baseNote = 72; componot=84; } // if (sx == 5) // { // baseNote = 48; // } // // if (sx == 6) // { // baseNote = 60; // } // // if (sx == 7) // { // baseNote = 72; // } // // if (sx == 8) // { // baseNote = 84; // } // // if (sx == 9) // { // baseNote = 96; // } Serial.print ( "Scale: "); Serial.println (sx); Serial.print ( " Value "); Serial.println (scale); // Here we read the values from the sensors digitalWrite(trigPin1, LOW); // Added this line delayMicroseconds(2); // Added this line digitalWrite(trigPin1, HIGH); delayMicroseconds(10); // Added this line digitalWrite(trigPin1, LOW); duration1 = pulseIn(echoPin1, HIGH); distance1 = (duration1) / 29.1; dx = map(distance1, 0, 60, 1, 13); Serial.print ( "Sensor1 "); Serial.print ( distance1); Serial.println("cm"); Serial.print ( " Value"); Serial.println ( dx); delay(500); digitalWrite(trigPin2, LOW); // Added this line delayMicroseconds(2); // Added this line digitalWrite(trigPin2, HIGH); delayMicroseconds(10); // Added this line digitalWrite(trigPin2, LOW); duration2 = pulseIn(echoPin2, HIGH); distance2 = (duration2) / 29.1; dy = map(distance2, 0, 60, 1, 13); Serial.print ( "Sensor2 "); Serial.print ( distance2); Serial.println("cm"); Serial.print ( " Value"); Serial.println ( dy); delay(200); // By the distance, the note will be asssigned and the message sended with the specific note depending on the distance and assigned to each sensor reading if (dx != lastDistance ) { delay(5); // debounce delay if (dx == 1) { note = note; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 2) { note = note + 2; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 3) { note = note + 4; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 4) { note = note + 5; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 5) { note = note + 7; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 6) { note = note + 9; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 7 ) { note = note + 11; lastnote = note; midiCommand(0x90, note, 127); } if (dx == 8) { note = note + 12; lastnote = note; midiCommand(0x90, note, 127); } // if (dx == 9) { // note = note + 8; // lastnote = note; // midiCommand(0x90, note, 127); // // } // if (dx == 10) { // note = note + 9; // lastnote = note; // midiCommand(0x90, note, 127); // // } // // if (dx == 11) { // note = note + 10; // lastnote = note; // midiCommand(0x90, note, 127); // // } // // if (dx == 12) { // note = note + 11; // lastnote = note; // midiCommand(0x90, note, 127); // // } } else { midiCommand(0x80, lastnote, 0); } lastDistance = dx; lastnote = note; midiCommand(0x80, lastnote, 0); if (dy != lastDistance1 ) { delay(5); // debounce delay if (dy == 1) { comp = componot; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 2) { comp = componot+2; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 3) { comp = componot+4; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 4) { comp = componot + 5; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 5) { comp = componot + 7; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 6) { comp = componot + 9; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 7 ) { comp = componot + 11; lastnote2 = comp; midiCommand(0x90, comp, 127); } if (dy == 8) { comp = componot+ 12; lastnote2 = comp; midiCommand(0x90, comp, 127); } // if (dx == 9) { // note = note + 8; // lastnote = note; // midiCommand(0x90, note, 127); // // } // if (dx == 10) { // note = note + 9; // lastnote = note; // midiCommand(0x90, note, 127); // // } // // if (dx == 11) { // note = note + 10; // lastnote = note; // midiCommand(0x90, note, 127); // // } // // if (dx == 12) { // note = note + 11; // lastnote = note; // midiCommand(0x90, note, 127); // // } } else { midiCommand(0x80, lastnote2, 0); } lastDistance1 = dy; lastnote2 = comp; midiCommand(0x80, lastnote2, 0); } //Function to send midi Note Message void midiCommand(byte cmd, byte data1, byte data2) { // First parameter is the event type (top 4 bits of the command byte). // Second parameter is command byte combined with the channel. // Third parameter is the first data byte // Fourth parameter second data byte midiEventPacket_t midiMsg = {cmd >> 4, cmd, data1, data2}; MidiUSB.sendMIDI(midiMsg); }
OUR NEXT STEPS / CONCLUSIONS
In future we'd like to make it work like the harmonic circle so it's easier to compose music.
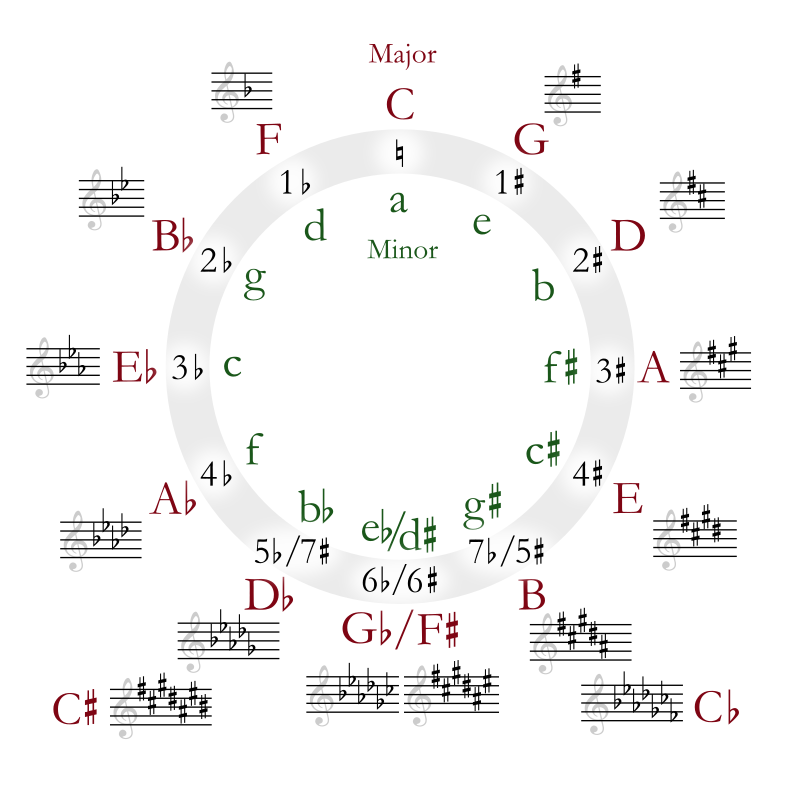
Comments